TL;DR
This post we are going to down the core concepts of programming, focusing on key principles like variables, data types, control flow, functions, and algorithms. By mastering these foundational concepts, beginners can build strong coding skills and gain the confidence to tackle more complex challenges. The guide emphasizes the importance of understanding these basics, as they are essential for writing efficient, readable code and approaching problems logically, setting the stage for success in programming.
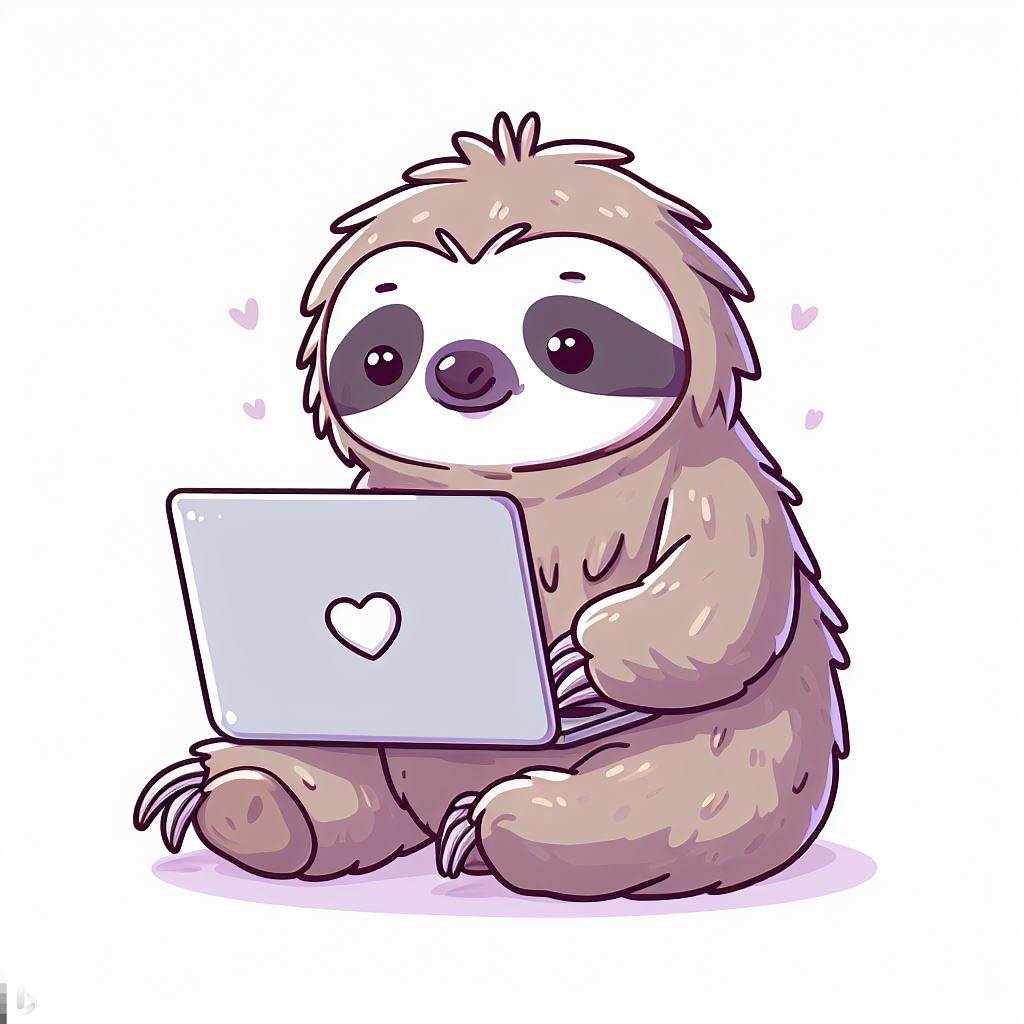
Cost, Code, and Calculation: Understanding Variables Through a Safari Adventure
Learn how to solve a high school-level math problem step by step, then explore how variables in programming can help tackle the same problem more efficiently.
Field Trip Math: A Safari Adventure
We are going to solve a high school-level math problem by breaking down the costs of a field trip to a safari park. As we work through the math, we’ll approach it step by step just like you would in any high school math class. Once we’ve completed the calculations, we’ll explore how variables in programming can help us solve the same problem in a more efficient way. Let’s get started!
Problem:
A group of 10 students went on a field trip to Safari Adventure Park. The total cost of the trip was divided into three categories:
Transportation: $200
Lunch: $120
Ticket cost per student: $9.50
The total cost for the trip is shared equally among all the students.
Question:
What is the total cost of the trip, and how much does each student contribute?
Solution:
Step 1: Identify the key information given:
Park name: Safari Adventure Park
Number of students: 10
Transportation cost: $200
Lunch cost: $120
Ticket cost per student: $9.50
Step 2: Calculate total Total ticket cost
Total ticket cost = Number of students × Admission ticket cost per student
= 10 × $9.50
= $95.0
Step 3: Calculate total trip cost
Total cost of the trip = Transportation cost + Lunch cost + Total ticket cost
= $200 + $120 + $95.0
= $415
Step 4: Calculate contribution by each student
Contribution by each student = Total cost / Number of students
= $415 / 10
= 41.5
We have solved this math step by step. In the next section we will solve this problem using Python, which is popular programing language and its easier for beginners to get started with.
Learning Variables Through a Safari Adventure
For the time being we will not use any space ” ” to store information as we did in the earlier section to solve the math. Instead of spaces ” ” we will use a underscore “_”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
# Step 1: Identify the key information park_name = "Safari Adventure Park" number_of_students = 10 transportation_cost = 200 lunch_cost = 120 ticket_cost_per_student = 9.50 # Step 2: Calculate the total ticket cost total_ticket_cost = number_of_students * ticket_cost_per_student # Step 3: Calculate the total trip cost total_trip_cost = transport_cost + lunch_cost + total_ticket_cost # Step 4: Calculate the contribution by each student contribution_per_student = total_trip_cost / number_of_students # Output the results print(f"Total trip cost for the group: ${total_trip_cost}") print(f"Contribution by each student: {contribution_per_student}") |
Expected Output of the program
1 2 |
Total trip cost for the group: $415.0 Contribution by each student: $41.5 |
To solve this math problem we have used few data types.
String (str):Example:
park_name = “Safari Adventure Park”
Integer (int):
Example: number_of_students = 10
transportation_cost = 200
lunch_cost = 120
Float (float)
Example: ticket_cost_per_student = 9.50
contribution_per_student = total_trip_cost / number_of_students
Introduction:Why Core Concepts Matter in Coding
Coding can seem like an overwhelming task, especially when you’re just starting out. With numerous programming languages, frameworks, and libraries to choose from, it’s easy to feel lost. However, at the heart of every programming language, there are a few fundamental concepts that all coders, no matter the language or tool, need to understand. In this post, we’ll break down these core concepts, explore why they are essential, and how mastering them will lay a strong foundation for your coding journey. By understanding these fundamentals, you’ll gain clarity, confidence, and the ability to approach any coding challenge with a systematic mindset.
While Artificial Intelligence (AI) can be a helpful tool to assist in writing code, it’s crucial to understand the basics first. AI-powered coding assistants, like GitHub Copilot or ChatGPT, can speed up your work and help you solve problems faster, but without a solid grasp of core concepts, you might struggle to effectively use these tools. AI is not a replacement for understanding programming fundamentals—it’s a powerful supplement that enhances your productivity once you’ve built a strong foundation. Let’s dive into the essential concepts every coder should know to master programming and harness the power of AI tools effectively.
1. Variables and Data Types
What are Variables?
Variables are like containers where you can store data that your program can use. In simple terms, a variable holds a value, such as a number, a string (text), or a boolean (true/false).
Why Are They Important?
Variables allow programs to store and manipulate data. Whether you’re building a simple calculator or an advanced machine learning model, you’ll always need to use variables to hold values and track changes over time.
Common Data Types:
Integers (int) – Whole numbers like 1, -5, or 42
Floats (float) – Decimal numbers like 3.14 or -0.01
Strings (str) – Text values like “hello world” or “John”
Booleans (bool) – True or False values
2. Control Flow: Making Decisions in Code
What is Control Flow?
Control flow refers to the order in which the instructions in your program are executed. It’s what allows your program to make decisions and perform actions based on certain conditions.
Key Concepts:
Conditionals – Using if, else, and elif (in Python) to make decisions.
Loops – Repeating a block of code a certain number of times using for or while loops.
Why Control Flow is Crucial:
Without control flow, your program would run line by line without any decision-making capabilities. Mastering control flow helps you create dynamic, responsive programs that behave intelligently.
3. Functions: Reusable Code Blocks
What are Functions?
Functions are blocks of code designed to perform a specific task. Once a function is defined, it can be used multiple times in different parts of your code without needing to rewrite the same code over and over again.
Why Are Functions Important?
Functions help make code more modular and organized. They also allow for code reuse, making programs more efficient and easier to maintain. Functions can accept inputs (parameters) and return outputs (return values).
4. Arrays (or Lists) and Collections: Storing Multiple Values
What are Arrays or Lists?
An array (or list, depending on the language) is a collection of elements, where each element is a value stored in an ordered sequence. For example, a list of numbers: [1, 2, 3, 4, 5].
Why Are Arrays Important?
Arrays allow you to store and manipulate large collections of data, such as all users in a system, items in a shopping cart, or scores in a game. Learning how to use arrays efficiently is essential for handling data in coding.
5. Object-Oriented Programming (OOP)
What is OOP?
Object-Oriented Programming is a programming paradigm that uses “objects” to represent real-world entities and concepts. In OOP, classes are blueprints for creating objects, and objects contain both data (attributes) and behaviors (methods).
Why is OOP Important?
OOP helps structure complex programs by grouping related data and behavior into objects. It promotes code reuse, scalability, and maintenance. The four main principles of OOP are:
Encapsulation – Bundling data and methods inside a class.
Abstraction – Hiding the internal workings of a class while exposing only the essential details.
Inheritance – Creating new classes based on existing ones.
Polymorphism – Allowing different objects to be treated as instances of the same class through a common interface.
6. Algorithms and Problem-Solving
What are Algorithms?
Algorithms are step-by-step instructions used to solve a problem or perform a task. Coding is largely about developing and optimizing algorithms to achieve the desired results efficiently.
Why are Algorithms Important?
Having a strong grasp of algorithms is crucial for solving problems effectively, whether you’re sorting data, searching for a specific item, or processing a large dataset. Algorithms also play a key role in the performance and speed of your code.
7. Debugging and Error Handling
What is Debugging?
Debugging is the process of identifying and fixing errors in your code. Whether it’s a simple typo or a more complex logical error, debugging is an essential skill every coder must develop.
Why is Debugging Important?
No matter how skilled you become, errors are inevitable. The ability to debug efficiently will save you time, reduce frustration, and make you a more effective coder. Learning error-handling techniques like try-except (in Python) or try-catch (in Java) can also help manage unexpected situations gracefully.
8. Understanding Data Structures
What Are Data Structures?
Data structures are ways of organizing and storing data so that it can be accessed and modified efficiently. Common data structures include arrays, linked lists, stacks, queues, and trees.
Why Are Data Structures Important?
Choosing the right data structure is crucial for optimizing the performance of your programs. For example, using a hash map (dictionary in Python) allows for faster lookups compared to a list.
9. Libraries and Frameworks
What Are Libraries and Frameworks?
Libraries are collections of pre-written code that help simplify tasks, like file handling, networking, or data processing. Frameworks provide a structure for developing applications, guiding you through the coding process.
Why Are They Important?
Instead of reinventing the wheel, libraries and frameworks allow you to focus on what’s unique about your project. They save time and effort while making your code more robust and scalable.
10. Version Control with Git
What is Git?
Git is a version control system that allows multiple developers to work on the same codebase simultaneously. It tracks changes, helps merge work, and provides backup versions of the project.
Why is Git Important?
Understanding Git and platforms like GitHub or GitLab is crucial for collaboration and ensuring that your project is organized and safe from potential data loss. Version control is especially important for working on larger projects or with teams.
11. Time and Resource Management
What is Time and Resource Management in Coding?
Coding can be time-consuming, and managing your time and resources effectively is key to avoiding burnout. This involves prioritizing tasks, breaking down large projects into smaller tasks, and knowing when to ask for help.
Why is Time Management Important?
Good time management helps you avoid procrastination and stay on track to meet deadlines. By managing your resources well, you can become more productive and efficient in your coding journey.
Conclusion
Mastering the Core Concepts
Mastering the core concepts of coding is essential for becoming a proficient programmer. These fundamental building blocks, such as variables, data structures, algorithms, and control flow, are not only the backbone of coding but will also empower you to tackle more complex problems and projects as you progress.
Remember, coding is a journey, and understanding these core concepts will make it much easier to navigate. With practice, patience, and persistence, you’ll find yourself moving from beginner to expert in no time.