TL;DR
Practice simple coding problems regularly , it is key to improving your programming skills. Start with basic problems, gradually progress to more complex ones, and prepare for coding interviews along the way.
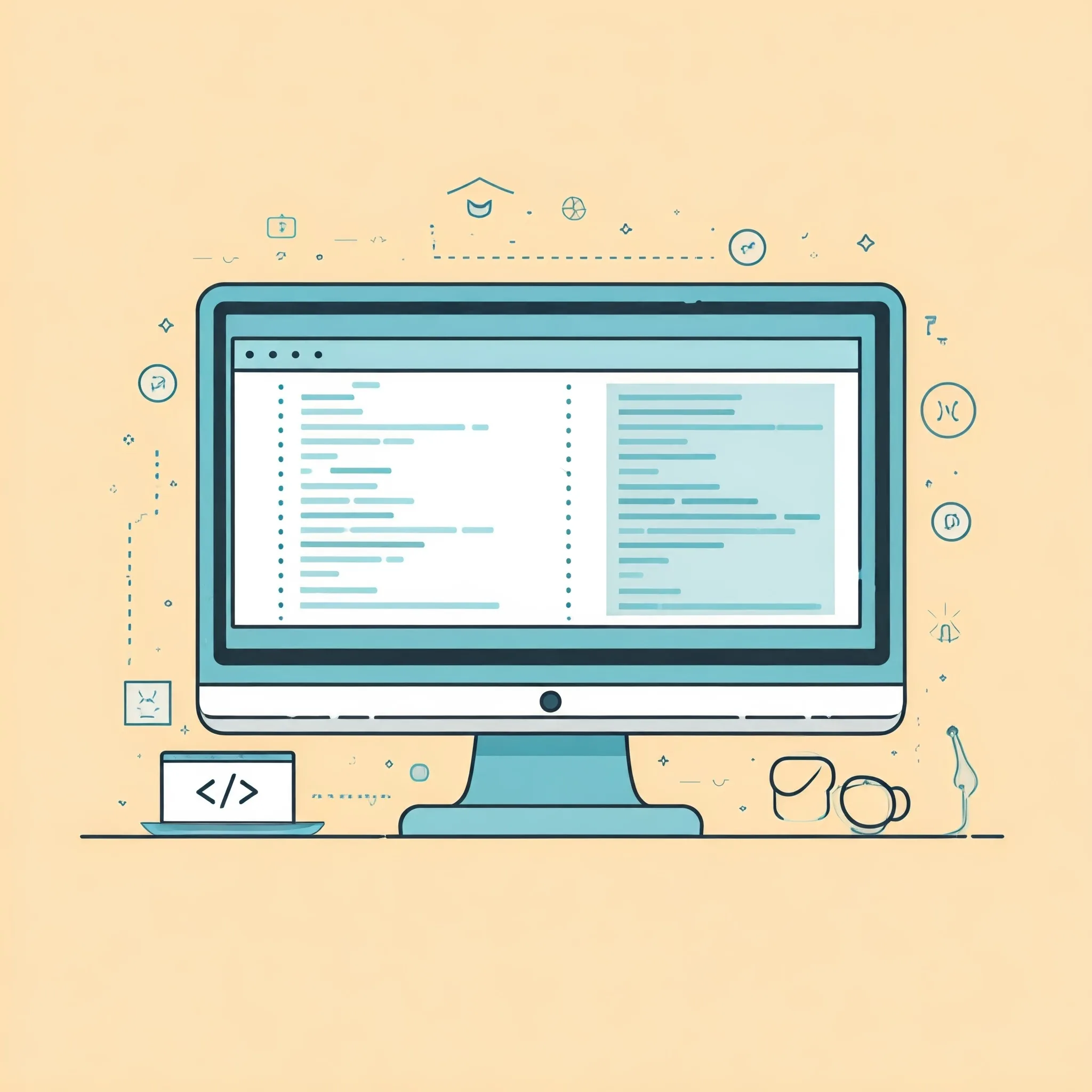
Introduction:
When you begin your journey into programming, the road can often feel overwhelming. There’s so much to learn—different languages, frameworks, algorithms, and development tools. One of the most effective ways to build your skills and boost your confidence is by practicing simple coding problems regularly. These seemingly small tasks can help you master fundamental concepts, improve your problem-solving abilities, and set a solid foundation for tackling more complex challenges.
In this blog, we’ll explore why practicing simple coding problems is essential for beginners and how this habit can fast-track your programming progress.
Why Practice Simple Coding Problems?
1. Builds a Strong Foundation
Before diving into complex projects, it’s crucial to understand the fundamentals. Simple coding problems help reinforce your understanding of basic concepts like variables, loops, conditionals, functions, and data structures. By regularly practicing these small tasks, you’ll develop a solid foundation that makes it easier to tackle more advanced topics in the future.
2. Improves Problem-Solving Skills
Programming isn’t just about writing code—it’s about solving problems efficiently. Simple coding problems teach you how to break down larger problems into smaller, more manageable steps. This strengthens your problem-solving skills, allowing you to think critically and logically when facing more complicated challenges.
3. Increases Coding Speed and Confidence
The more you practice, the faster you become. Coding regularly helps you get comfortable with syntax and problem-solving patterns, which in turn increases your speed and accuracy. Over time, solving problems will feel more intuitive, and you’ll develop a higher level of confidence in your abilities.
4. Provides a Sense of Accomplishment
Completing coding problems, no matter how small, gives you a sense of achievement. This positive reinforcement motivates you to keep learning and tackling harder challenges. It’s also a great way to track your progress and see how far you’ve come over time.
5. Prepares You for Interviews and Competitions
If you’re aiming for a career in tech or software development, practicing simple coding problems can be especially helpful in preparing for coding interviews. Many tech companies use algorithm and data structure problems as part of their interview process. Regular practice will not only help you get familiar with common problem types but also sharpen your coding and problem-solving skills under time constraints. Additionally, platforms like LeetCode, HackerRank, and Codewars provide excellent resources for coding competitions.
How to Practice Simple Coding Problems Effectively
1. Start with the Basics
When you’re just starting out, focus on the basics. Solve problems related to:
Variables and data types
Loops and conditionals
Functions and recursion
Arrays and strings
Checkout more on the basics
Examples:
Palindrome Checker: Write a program that checks if a string is a palindrome.
FizzBuzz: Print numbers from 1 to 100, but replace multiples of 3 with “Fizz” and multiples of 5 with “Buzz.”
These are excellent warm-up exercises for getting comfortable with basic syntax and logic.
2. Gradually Increase the Difficulty
As you become more comfortable with simpler problems, start introducing more complex topics, such as:
Sorting and searching algorithms (e.g., Bubble Sort, Merge Sort)
Working with hash maps or dictionaries
Linked lists, stacks, and queues
Recursion and dynamic programming
While these topics may seem challenging at first, taking small steps and solving related problems will help you build the necessary skills over time.
3. Use Online Platforms
There are numerous online platforms that offer a wide range of coding problems suited for different skill levels. Some of the most popular ones include:
LeetCode: A favorite for preparing for technical interviews with a vast collection of coding challenges.
HackerRank: Offers practice problems categorized by difficulty, topic, and company.
Codewars: A community-driven platform where you can solve challenges (“katas”) and earn ranks.
Exercism.io: Provides coding exercises in multiple programming languages.
These platforms allow you to practice at your own pace and track your progress over time.
W3Schools: A comprehensive online resource offering tutorials and references on web development technologies like HTML, CSS, JavaScript, Python, and more.
4. Join Coding Communities
Join programming communities or online groups where you can discuss problems, share solutions, and learn from others. Platforms like Stack Overflow, Reddit’s Learn Programming community, or GitHub are great for connecting with other learners and experienced developers.
5. Set Aside Time for Regular Practice
Consistency is key when it comes to improving your coding skills. Set aside dedicated time each day or week to work on coding problems. Even solving just one problem a day will add up over time, and the practice will start to feel like second nature.
3. Examples of Simple Coding Problems to Start With
Here are a few examples of simple coding problems that are great for beginners:
- Reverse a String
Write a program that reverses a given string. - Find the Largest Number in an Array
Write a function that takes an array of numbers and returns the largest number. - Count the Vowels in a String
Create a program that counts how many vowels (a, e, i, o, u) are in a string. - Sum of Even Numbers
Write a program that takes an array of numbers and returns the sum of all even numbers. - Prime Number Check
Create a function that checks if a number is prime. - Check for Palindrome
Write a function that checks if a given string is a palindrome (reads the same forwards and backwards). - Factorial of a Number
Create a program that calculates the factorial of a number. - Fibonacci Sequence
Write a function that returns the nth number in the Fibonacci sequence. - Find the Smallest Number in an Array
Write a function that finds and returns the smallest number in a given array of numbers. - Sum of Digits in a Number
Write a program that takes a number and returns the sum of its digits. - Count Occurrences of a Character
Write a program that counts how many times a particular character appears in a string. - Check for Anagram
Create a function that checks if two strings are anagrams (i.e., they contain the same characters in any order). - Find the GCD of Two Numbers
Write a function that calculates the greatest common divisor (GCD) of two numbers. - Check if a Number is Even or Odd
Write a program that checks whether a given number is even or odd. - Sum of Odd Numbers
Write a function that calculates the sum of all odd numbers in an array. - Convert a String to Uppercase/Lowercase
Create a function that converts a string to all uppercase or all lowercase letters. - Find the Length of a String
Write a program that calculates the length of a given string without using built-in functions. - Print a Multiplication Table
Write a program that prints the multiplication table for a given number. - Reverse an Array
Create a program that reverses an array of numbers. - Merge Two Arrays
Write a function that merges two arrays into one, maintaining the order of the elements. - Check for Perfect Number
Create a function that checks if a number is a perfect number (a number that is equal to the sum of its divisors, excluding itself). - Convert a Decimal Number to Binary
Write a program that converts a given decimal number to binary. - Find the Common Elements in Two Arrays
Write a program that finds and returns the common elements in two arrays. - Remove Duplicates from an Array
Create a function that removes duplicate elements from an array. - Find the Most Frequent Element in an Array
Write a function that finds and returns the most frequent element in an array. - Check if a String is a Valid Number
Write a program that checks if a given string can be converted to a valid number. - Find the Second Largest Number in an Array
Create a function that finds and returns the second largest number in an array. - Count the Words in a Sentence
Write a function that counts the number of words in a given sentence. - Create a Pyramid Pattern
Write a program that prints a pyramid pattern of stars (or any character) with a specified number of rows. - Calculate the Average of Numbers in an Array
Write a program that calculates and returns the average of all numbers in a given array. - Dice Roll Simulator
Create a program that simulates the rolling of a 6-sided dice, picking a random number between 1 and 6. - Create a Simple Calculator
Write a program that can perform basic arithmetic operations (addition, subtraction, multiplication, division) based on user input. - Guess the Number Game
Create a game where the program picks a random number between 1 and 100, and the user has to guess the number. The program should give hints (higher/lower) after each guess. - Random Password Generator
Write a program that generates a random password containing uppercase, lowercase letters, digits, and special characters. - Number Guessing Game (With Attempts Limit)
Create a number guessing game where the user has a limited number of attempts to guess the correct number. - Leap Year Checker
Write a function that checks if a given year is a leap year or not. - Find Prime Numbers in a Range
Create a program that lists all prime numbers within a given range (e.g., 1 to 100). - Create a Countdown Timer
Write a program that starts a countdown from a specified number of seconds, displaying the countdown in real-time. - Check if a Word is a Pangram
Create a function that checks if a given sentence contains every letter of the alphabet at least once (pangram). - Sum of Multiples
Write a program that finds the sum of all multiples of a number (e.g., multiples of 3) within a given range. - Create a Simple To-Do List
Create a program that allows users to add, delete, and display tasks in a to-do list. - Temperature Converter
Write a program that converts temperatures between Celsius and Fahrenheit. - Find the Mode of a List
Create a function that finds the most frequent number in a list and returns the mode. - Build a Simple Countdown Timer
Write a program that counts down from a specific time (in seconds) and prints the time remaining. - Calculate the Sum of Prime Numbers in a Range
Write a program that sums up all prime numbers in a given range (e.g., 1 to 50). - Create a Digital Clock
Write a program that shows the current time on the console in the format “HH:MM:SS” and updates every second. - Matrix Multiplication
Create a function that multiplies two matrices and returns the result. - Check if a String Contains Only Digits
Write a program that checks if a string contains only digits and no other characters. - Create a Countdown Timer with User Input
Write a program that allows the user to input the time in seconds and then starts a countdown timer. - Find the Largest Palindrome in a List
Create a function that finds the largest palindrome in a list of words. - Print All Substrings of a String
Write a function that prints all possible substrings of a given string. - Create a Simple Alarm Clock
Write a program where the user can set an alarm (input a specific time), and the program will notify when the time arrives. - Reverse Words in a Sentence
Write a program that reverses the order of the words in a sentence without reversing the letters of each word. - Find Missing Number in an Array
Write a program that finds the missing number in an array of numbers from 1 to n (the array contains all numbers except one). - Create a Tic-Tac-Toe Game
Write a simple two-player Tic-Tac-Toe game that can be played in the terminal or console.
These problems may seem simple, but they require you to think critically about how to approach a solution and write clean, efficient code.
Conclusion
Practice simple coding problems regularly, it is one of the most effective ways to improve your programming skills. It helps you build a strong foundation, improves your problem-solving abilities, and boosts your confidence. Whether you’re a beginner or an experienced developer, there’s always value in revisiting the basics. So, start solving problems today and watch your coding skills grow!
Remember: Coding is a journey, not a race. The more consistent you are with your practice, the more you’ll see your progress. Happy coding!